@StrmCkr
first of all I want to say that I really appreciate your effort to help me.
Me too I will try to be as clear as possible in this post.
As already mentioned, I want to point out that my goal is not solving sudokus in general, I just enjoy implementing logic problems in C/C++, and sudoku is one of them.
Before looking on the internet I had already implemented the basic strategies, which I later learned are called: Naked Candidates, Hidden Candidates, Intersection Removal, Avoidable Rectangles, Unique Rectangles (basic cases), plus my own version of a brute force algorithm used when all resolution techniques fail.
At that point I started looking for advanced solving techniques and stumbled upon the SudokuWiki site, which I found well done.
As already mentioned, however, I have often used it only to understand the basic idea of the various resolution techniques (in some cases I have only looked at the images, also considering my difficulties with english), to then build my own personal logic approach and algorithm. This is also what happened with the x-cycles, in fact the concepts of "alternating strong_link-weak_link", "discontinuity" and "strong links acting as weak links" are completely absent in my approach and were not necessary for my problem modeling, which I report for convenience:
Mino21 wrote:a) only two candidates in an unit imply a strong link and hence the following two logical implications:
- Code: Select all
A => !B
!A => B
more than two candidates in a unit imply a weak link and hence the only following logical implication:
- Code: Select all
A => !B
b) a valid loop must have the following characteristics:
- at least 4 nodes;
- at least a weak link;
- at most an even subsequence of consecutive strong links or a subsequence of two consecutive weak links;
- (any number of odd subsequences of consecutive strong links).
c) loop rules (green=true and red=false; arrows indicate the starting point and the direction of the logical inferences):
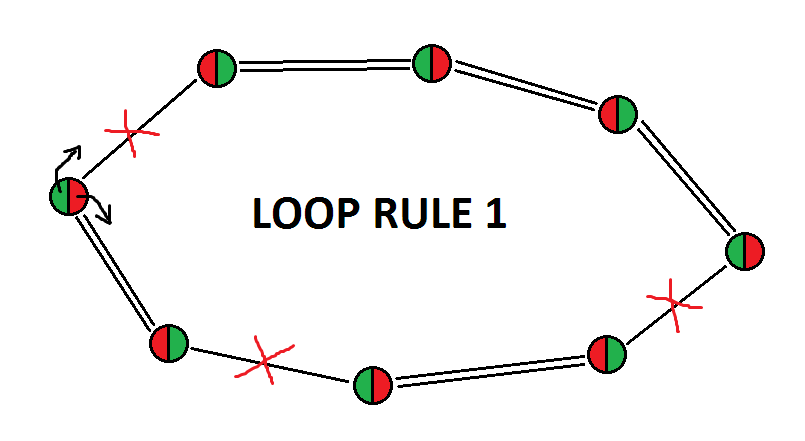
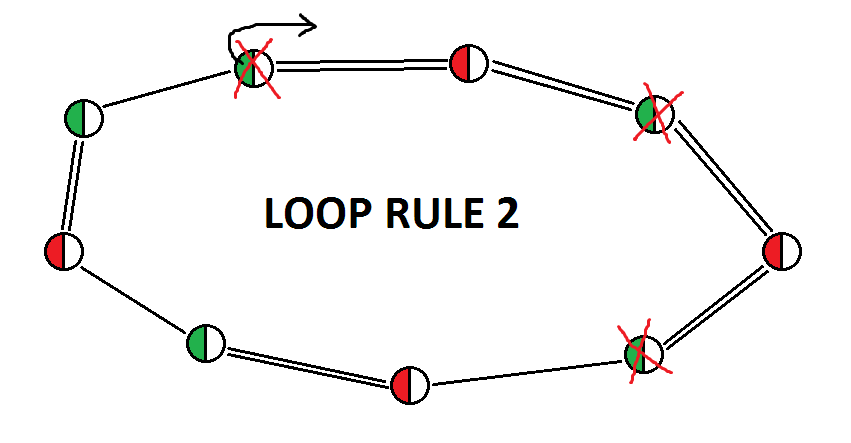
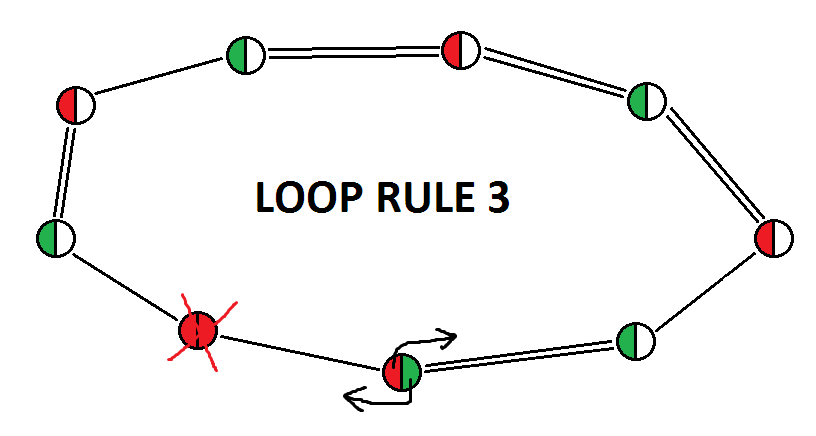
d) implemented recursive algorithm about x-cycles (not grouped):
- I always start from a strong link, of which a candidate will be the arrival node that closes the loop, while I pass the other to the recursive function that, node by node, tries to build a loop;
- at each recursive call, I first use the information on the part of the loop constructed so far to deduce (using positions contained in point b)) if the next link can be weak or must necessarily be strong;
- then I derive the type of connection (weak or strong) that I would have in the other units to which the previous node belongs, and check that it is compatible with the result illustrated in the previous point;
- if it is compatible I consider the various candidates in that unit one at a time, and if the candidate considered is not already a node I add it to the cycle;
- at this point if the loop isn't closed I go on with other recursive calls.
with the specification that with grouped-x-cycles in the case of mini rows and mini columns that share a cell, it is necessary to consider the two cases obtained by assigning the common cell once to the horizontal group and once to the vertical one, as shown in the following image:
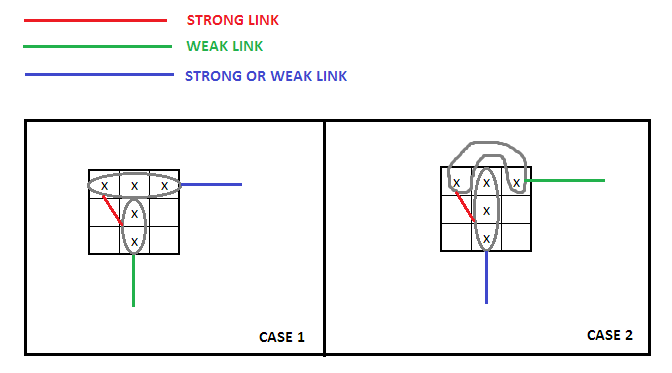
Let me clarify some points that may not be very clear:
- the demonstration of the loop rules (having used SudokuWiki as a source, by convention I used as names those reported in the aforementioned site) in point c) are a consequence of the positions contained in points a) and b).
In particular:
loop rule 1 is characterized by:
1) at least one weak link;
2) no subsequence of two consecutive weak links;
3) no even subsequence of consecutive strong links;
4) any number of odd subsequences of consecutive strong links;
5) even number of nodes (but this is a consequence of the previous points)
Proof: starting from a node belonging to a weak link, assuming once it is true and once it is false, and using the logical inferences contained in point a), we will obtain two perfectly mirror chains, which will allow us to eliminate the candidates in the unit to which the weak links belong (of course I am referring to the cells that are not nodes).
loop rule 2 is characterized by:
1) at least one weak link;
2) no subsequence of two consecutive weak links;
3) one even subsequence of consecutive strong links;
4) any number of odd subsequences of consecutive strong links;
5) odd number of nodes (but this is a consequence of the previous points)
Proof: starting from the node that starts the even subsequence of consecutive strong links, and assuming it is true, we will obtain a contradiction, which will allow us to eliminate the candidates in the nodes marked as true and that belong to the even subsequence of consecutive strong links.
loop rule 3 is characterized by:
1) at least one weak link;
2) one subsequence of two consecutive weak links;
3) no even subsequence of consecutive strong links;
4) any number of odd subsequences of consecutive strong links;
5) odd number of nodes (but this is a consequence of the previous points)
Proof: starting from the node that starts the subsequence of two consecutive weak links, and assuming once it is true and once it is false, we will obtain that the node that sees two weak links is false in both cases, which will allow us to eliminate the candidate in it;
- when a mini-row and a mini-column have a cell in common as in this case:
- Code: Select all
---------
| x x x |
| / x / |
| / x / |
---------
I cannot consider the strong link r1c123=r123c2 cause if r1c2 is solution then the logic inference A=>!B, reported at point a), is not respected. This is the reason why I split r1c123=r123c2 in r1c123=r23c2 and r1c13=r123c2.
And with this I believe and hope to have clarified everything that needed to be clarified.
Now let's move on to the various candidates configurations you posted:
StrmCkr wrote:- Code: Select all
-------------------------
| X X X | . . . |-X-X / |
| / X / | . . . | . . / |
| /-X / | . . . | . . x |
-------------------------
| . . . | . . . | . . / |
| . . . | . . . | . . / |
| . . . | . . . | . . / |
-------------------------
| / x / | . . . | . . / |
| / X / | . . . | . . / |
| x x x | . . . | . . x |
-------------------------
I try to explain how my algorithm works in this case:
- as mentioned in point d), I always start from a strong link, for example the one in c9, and let r9c9 be the arrival node and r3c9 the starting node;
- from the data on the part of the cycle constructed so far, I deduce that the next link can also be weak (ALSO WEAK) and not necessarily strong (ONLY STRONG);
- considering all the possible connections from r3c9, at a certain point I add the node r1c78 with a weak link (-r1c78);
...going on with the notation introduced in the previous two lines...
- ALSO WEAK;
- -r1c123;
- ONLY STRONG;
- =r23c2;
- ALSO WEAK;
- -r78c2;
- ONLY STRONG;
- =r9c123;
- ALSO WEAK;
- -r9c9;
- the chain is closed, I check loop is valid and I deduce that it is a loop rule 3 that delete candidates in r1c78.
To sum up:
r9c9=r3c9-r1c78-r1c123=r23c2-r78c2=r9c123-r9c9
L.R.3 (loop rule 3): r1c78 (elimination)
The aforementioned cycle does not allow me to delete candidate in r3c2, but this other cycle yes:
r9c9=r3c9-r3c2-r789c2=r9c13-r9c9
L.R.3: r3c2
StrmCkr wrote:- Code: Select all
-------------------------
| X X X | . . . |-X-X / |
| / X / | . . . | . . / |
| /-X / | . . . | . . x |
-------------------------
| . / . | . . . | . . / |
| . / . | . . . | . . / |
| . / . | . . . | . . / |
-------------------------
| / x / | . . . | . . / |
| / X / | . . . | . . / |
| x x x | . . . | . . x |
-------------------------
r9c9=r3c9-r1c78-r1c123=r23c2-r78c2=r9c123-r9c9
L.R.3: r1c78
r9c9=r3c9-r3c2-r789c2=r9c13-r9c9
L.R.3: r3c2
StrmCkr wrote:- Code: Select all
-------------------------
| X X X | . . . | .-x . |
| / X / | . . . | . . . |
| / X / | . . . | . . . |
-------------------------
| . . . | . . . | . . . |
| . . . | . . . | . . . |
| . . . | . . . | . . . |
-------------------------
| / x / | . . . | / x / |
| X X X | . . . | x x x |
| / x / | . . . | / x / |
-------------------------
r8c123-r8c789=r79c8-r1c8-r1c123=r23c2-r79c2=r8c123
L.R.3: r1c8
About the last two examples with 1 you posted I did not understand what they represent.
So in the end my approach seems to work with all the examples you posted, am I wrong?
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
P.O. wrote:hi Mino21, my reading of the diagram:
if r123c2 is not x then r2c8 is x then r9c13 is not x then one of r9c79 is x: it is not known which one
if r123c2 is not x then one of r1c13 is x then r1c7 is not x then one of r2c7 r3c9 is x it is not known which one
in either case the loop can't be built
Hi P.O., if you're referring to
this, we can built the following loop:
r123c2=r8c2=r9c13-r9c9=r3c9-r1c7-r1c13=r123c2
So we have a loop rule 3 with elimination in r1c7.
----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
creint wrote:Is your solver public somewhere (Mino21)?
No, I still have to fix various things.
Anyway, given that I have not yet studied AICs and other advanced strategies, in this message I have explained how I have implemented x-cycles.